6. Subsystem "Transports" (TTransportS)
"Transports" subsystem is represented by the TTransportS object, which contains modular objects of the transports' types TTypeTransport on the subsystem-level. Each type of transport contains objects TTransportIn of the incoming and TTransportOut of the outgoing transports. The overall structure of the subsystem is shown in Fig. 6.
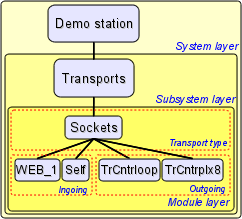
Fig. 6. The layered structure of the transports subsystem.
The root object of the "Transports" subsystem's module provides information about the specific type of module and about the external OpenSCADA hosts/stations. As part of the single module it can be implemented the own general-module functionality. In general, for all modules, the access methods for both: inbound and outbound transports of the specific module are contained.
The object of the incoming transport TTransportIn provides an interface to the implementation of the modular method of incoming transport.
The object of the outgoing transport TTransportOut provides an interface to the implementation of the modular method of outgoing transport.
6.1. The object of the "Transports" subsystem (TTransportS)
Data:
External hosts mode (enum — ExtHost::Mode):
- User (0) — User.
- System (1) — System.
- UserSystem (2) — User and System.
The structure of the external OpenSCADA hosts/stations (class TTransportS::ExtHost):
- ExtHost( const string &iUserOpen, const string &iid, const string &iname = "", const string &itransp = "", const string &iaddr = "", const string &iuser = "", const string &ipass = "", uint8_t iUpRiseLev = 0 ); — Constructor of the structure initialization.
- string userOpen; — User who created the record about the external host/station.
- string id; — Id of the external host/station.
- string name; — Name of an external host/station.
- string transp; — Transport which is used to access an external host/station.
- string addr; — Address for the transport, which is used to access an external host/station.
- string user; — Users of the external host/station.
- string pass; — Password of the external host/station user.
- uint8_t upRiseLev; — External hosts uprising level/depth of this host/station.
- int8_t mode; — Host mode.
- time_t mdf; — Modification time.
Public methods:
- int subVer( ); — Subsystem's version.
- void inTrList( vector<string> &ls ); — Full list of incoming transports.
- void outTrList( vector<string> &ls ); — Full list of outgoing transports.
- string extHostsDB( ); — Database for the storing the list of external hosts.
- void extHostList( const string &user, vector<ExtHost> &list, bool andSYS = false, int upRiseLev = -1 ); — The list of external hosts for user, include system andSYS and the uprising level upRiseLev (-1 - starting from the host's entry level).
- ExtHost extHostGet( const string &user, const string &id, bool andSYS = false ); — Getting the information object from the external host id on behalf of the user user, include system andSYS ( "*" — for the system hosts).
- AutoHD<TTransportOut> extHost( TTransportS::ExtHost host, const string &pref = "" ); — Creation - request of the outgoing transport to service the external host host with the prefix of the identification of the system node pref.
- void extHostSet( const ExtHost &host, bool andSYS = false ); — Setting of the external host/station host, include system andSYS.
- void extHostDel( const string &user, const string &id, bool andSYS = false ); — Deleting of the external host/station id on behalf of the user user, include system andSYS ( "*" — for the system hosts).
- int cntrIfCmd( XMLNode &node, const string &senderPref, const string &user = "" ); — Transfer of the control area request of the OpenSCADA node to the remote station.
- void subStart( ); — Start of the subsystem.
- void subStop( ); — Stop of the subsystem.
- TElem &inEl( ); — DB structure of the incoming transports
- TElem &outEl( ); — DB structure of the outgoing transports
- AutoHD<TTypeTransport> at( const string &id ) const; — Addressing/connection to the type of transport id.
6.2. The modular object of the transports' type (TTypeTransport)
Inherits: | TModule. |
Inherited: | By the root object of the subsystem's "Transports" modules.. |
Public methods:
- void inList( vector<string> &list ) const; — The list of the incoming transports.
- bool inPresent( const string &name ) const; — Check for an incoming transport presence.
- void inAdd( const string &name, const string &db = "*.*" ); — Addition of the incoming transport.
- void inDel( const string &name, bool complete = false ); — Deleting of the incoming transport. It is possible to completely delete with the database included, by setting the complete sign.
- AutoHD<TTransportIn> inAt( const string &name ) const; — Connection to the incoming transport.
- void outList( vector<string> &list ) const; — The list of the outgoing transports.
- bool outPresent( const string &name ) const; — Check for an outgoing transport presence.
- void outAdd( const string &name, const string &db = "*.*" ); — Addition of the outgoing transport.
- void outDel( const string &name, bool complete = false ); — Deleting of the outgoing transport. It is possible to completely delete with the database included, by setting the complete sign.
- AutoHD<TTransportOut> outAt( const string &name ) const; — Connection to the outgoing transport.
- TTransportS &owner( ) const; — "Transports" subsystem - the owner of the transport's type.
Protected methods:
- virtual TTransportIn *In( const string &name, const string &db ); — The modular method of the creating/opening of the new "incoming" transport.
- virtual TTransportOut *Out( const string &name, const string &db ); — The modular method of the creating/opening of the new "outgoing" transport.
6.3. The object of the incoming transports (TTransportIn)
Inherits: | TCntrNode, TConfig. |
Inherited: | By the objects of incoming transports of the subsystem's "Transports" modules. |
Public methods:
- TTransportIn( const string &id, const string &db, TElem *el ); — Initializing constructor.
- string id( ); — Transport's Id.
- string workId( ); — Full ID including the ID of the module.
- string name( ); — Transport's name.
- string dscr( ); — Transport's description.
- string addr( ) const; — Address.
- string protocol( ); — Linked transport protocol.
- virtual string getStatus( ); — Getting the status of the incoming transport.
- bool toStart( ); — The sign "To start".
- bool startStat( ); — The status "Running".
- string DB( ); — Transport's DB address.
- string tbl( ); — Transport's DB table.
- string fullDB( ); — The full name of the transport's DB table.
- void setName( const string &inm ); — Setting the name of transport in inm.
- void setDscr( const string &idscr ); — Setting the description of transport in idscr.
- void setAddr( const string &addr ); — Setting the address of transport in addr.
- void setProtocol( const string &prt ); — Setting of the linked transport protocol.
- void setToStart( bool val ); — Setting of the sign "To start".
- void setDB( const string &vl ); — Setting of the transport's DB address.
- virtual void start( ); — Start of the transport.
- virtual void stop( ); — Stop of the transport.
- virtual int writeTo( const string &sender, const string &data ); — Sending data backward to the sender.
- vector<AutoHD<TTransportOut> > assTrs( bool checkForCleanDisabled = false ); — The assigned output transports massive, created by the input transport for typical requests to the connection initiator. Set checkForCleanDisabled for prior checking and clean up disabled ones.
- int logLen( ); — length of the IO log.
- void setLogLen( int vl ); — set length of the IO log.
- void pushLogMess( const string &vl ); — push the message vl to the log in format "{Message}\n{BinData}".
- TTypeTransport &owner( ) const; — Transport's type – the owner of the incoming transport.
Protected methods:
- string assTrO( const string &addr ); — The assigned output transport creation with address addr.
Protected attributes:
- bool run_st; — The sign "Run".
6.4. The object of the outgoing transports (TTransportOut)
Inherits: | TCntrNode, TConfig. |
Inherited: | By the objects of outgoing transports of the subsystem's "Transports" modules. |
Public methods:
- TTransportOut( const string &id, const string &db, TElem *el ); — Initializing constructor.
- string id( ); — Transport's Id.
- string workId( ); — Full ID including the ID of the module.
- string name( ); — Transport name.
- string dscr( ); — Transport description.
- string addr( ) const; — Transport address.
- virtual string timings( ); — Transport timeouts.
- int prm1( ); — First backup parameter.
- int prm2( ); — Second backup parameter.
- bool toStart( ); — Sign "To start".
- bool startStat( ); — Status "Running".
- time_t startTm( ); — Status "Time of starting/connection".
- virtual string getStatus( ); — Getting the status of the transport.
- string DB( ); — Transport's DB address.
- string tbl( ); — Transport's DB table.
- string fullDB( ); — The full name of the transport's DB table.
- void setName( const string &inm ); — Setting the name of transport.
- void setDscr( const string &idscr ); — Setting the description of transport.
- void setAddr( const string &addr ); — Setting the address of transport.
- virtual void setTimings( const string &vl ); — Setting the transport timeouts.
- void setPrm1( int vl ); — Setting of the first backup parameter.
- void setPrm2( int vl ); — Setting of the second backup parameter.
- void setToStart( bool val ); — Setting of the sign "To start".
- void setDB( const string &vl ); — Setting of the transport's DB address.
- virtual void start( int time = 0 ); — Start of the transport with connection time time.
- virtual void stop( ); — Stop of the transport.
- virtual int messIO( const char *obuf, int len_ob, char *ibuf = NULL, int len_ib = 0, int time = 0 ); — Sending of the data over the transport. The waiting time time of the connection (in milliseconds). The time in negative disables the transport's request/respond mode and allow independent reading/writing to a buffer IO, with the reading timeout time in absolute.
- void messProtIO( XMLNode &io, const string &prot ); — Sending of the data in the XML tree in over the transport using the transport protocol prot.
- int logLen( ); — length of the IO log.
- void setLogLen( int vl ); — set length of the IO log.
- void pushLogMess( const string &vl ); — push the message vl to the log in format "{Message}\n{BinData}".
- TTypeTransport &owner( ) const; — Type of transport - the owner of outgoing transport.
- ResMtx &reqRes( ); — The requesting resource, needs to global lock into protocols with fragments receiving.
Protected attributes:
- bool run_st; — The sign "Running".